TikTok data scraping involves the automated process of extracting data from the TikTok platform. This data can include user profiles, video metadata, comments, hashtags, trending sounds, and various engagement metrics. By collecting and analyzing this data, organizations and individuals can gain valuable insights into user behavior, content performance, and emerging trends.
In this detailed guide, we’ll explain how to scrap TikTok data and most importantly, how to work with that scraped data. Let’s begin.
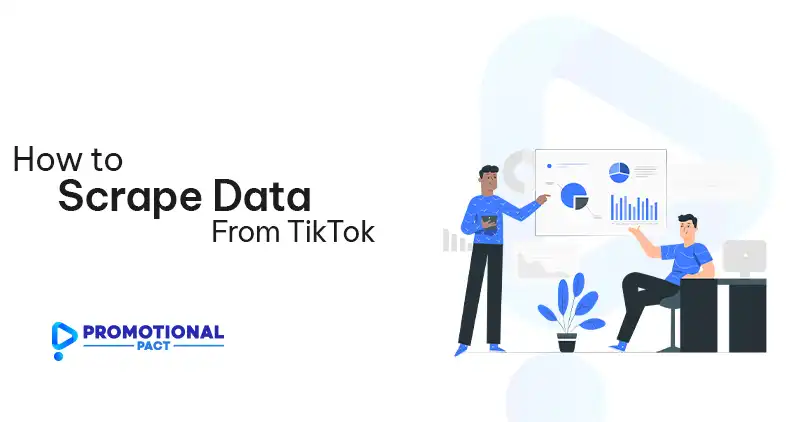
Scraping Data From TikTok: Official TikTok API vs. Web Scraping
When it comes to accessing TikTok data, there are two primary approaches: using the official TikTok Developer API or web scraping. Each method has its own advantages and limitations.
The official TikTok Developer API provides authorized access to certain data points, such as user profiles, videos, and hashtags. However, it has restricted access and may not offer all the data points you require for your specific use case.
Web scraping, on the other hand, involves extracting data directly from TikTok’s website or mobile app. While this approach can potentially provide more comprehensive data access, it raises ethical and legal considerations, as well as the risk of detection and potential consequences from TikTok.
Is It Legal to Scrape Data from TikTok
Before embarking on any TikTok data scraping endeavor, it’s crucial to understand and adhere to the legal and ethical guidelines.
TikTok’s Terms of Service and Scraping Guidelines
TikTok’s Terms of Service generally prohibit scraping activities that place an undue burden on their servers or disrupt the platform’s intended use. It’s essential to review TikTok’s guidelines and ensure compliance with their policies to avoid potential legal ramifications.
Leverage our data-driven influencer marketing solutions and leave the scraping to use and discover the most relevant and impactful creators for your brand on TikTok and beyond. Contact PromotionalPact today. |
Avoiding Copyright Infringement and Privacy Violations
When scraping TikTok data, it’s essential to respect intellectual property rights and user privacy. Avoid downloading or distributing copyrighted content, such as videos or audio, without proper authorization. Additionally, ensure that any personal user data collected is handled responsibly and anonymized when necessary.
Responsible Data Collection and Usage
Ethical data collection practices should be a top priority. Respect TikTok’s robots.txt guidelines, avoid overwhelming their servers with excessive requests, and use scraped data responsibly. Never engage in activities that could harm users or compromise the platform’s integrity.
Choosing Your Scraping Method
Based on your specific requirements and the level of data access needed, you’ll need to choose between using the official TikTok Developer API or web scraping with Python.
Using the Official TikTok Developer API
The official TikTok Developer API provides authorized access to certain data points, making it a viable option for some use cases.
1. Setting up a Developer Account and Applying for API Access
To access the TikTok Developer API, you’ll need to create a developer account on the TikTok for Developers platform. The application process involves providing details about your use case, intended data usage, and compliance with TikTok’s policies.
2. Exploring Available Endpoints (User Data, Hashtag Data, Video Statistics)
Once you’ve obtained API access, you can explore the available endpoints and data points. The TikTok API currently provides access to user data, hashtag data, video statistics, and more.
3. Making API Requests with Python (Authentication, Code Examples)
To interact with the TikTok API using Python, you’ll need to authenticate your requests and handle the API responses. Here’s a basic example of how to retrieve user data using the requests library:
import requests
# API endpoint and parameters
endpoint = "https://open.tiktokapis.com/user/info/"
params = {
"unique_id": "username",
"access_token": "your_access_token"
}
# Send the API request
response = requests.get(endpoint, params=params)
# Check if the request was successful
if response.status_code == 200:
user_data = response.json()
print(user_data)
else:
print("Error:", response.status_code)
4. Limitations of the TikTok API (Restricted Access, Limited Data Points)
While the official TikTok API provides authorized access, it has certain limitations. Access may be restricted based on your approved use case, and the available data points may not cover all your requirements. Additionally, rate limits and API costs can impact your data collection efforts.
Web Scraping with Python
Web scraping offers a more comprehensive approach to TikTok data collection, allowing you to extract data directly from the platform’s website or mobile app.
1. Choosing the Right Libraries (BeautifulSoup, Selenium)
Python provides various libraries for web scraping, each with its own strengths and use cases. BeautifulSoup is a popular library for parsing HTML and XML documents, making it suitable for scraping static content. Selenium, on the other hand, is a web automation tool that can handle dynamic content and interact with JavaScript-driven web applications, making it ideal for scraping TikTok’s modern web interface.
2. Building a Basic Scraper Script
To build a basic scraper script, you’ll need to navigate TikTok’s website or app, locate the relevant data elements, and extract the desired information.
Here’s an example of how to scrape TikTok user profiles using BeautifulSoup:
import requests
from bs4 import BeautifulSoup
# URL of the user profile page
url = "https://www.tiktok.com/@username"
# Send a request to the URL
response = requests.get(url)
# Parse the HTML content
soup = BeautifulSoup(response.content, "html.parser")
# Extract user data
username = soup.select_one("h2.share-title").text.strip()
bio = soup.select_one("h1.share-desc").text.strip()
follower_count = soup.select_one("h2.count-number").text.strip()
print("Username:", username)
print("Bio:", bio)
print("Follower Count:", follower_count)
a. Navigating Pages and Extracting Data
Web scraping often involves navigating through multiple pages or sections of a website to extract the desired data. This may require handling pagination, infinite scrolling, or dynamically loaded content.
b. Handling Pagination and Infinite Scrolling
Many websites, including TikTok, implement pagination or infinite scrolling to load content gradually. To scrape data from these pages, you’ll need to identify the patterns and simulate user behavior, such as scrolling or clicking on “Load More” buttons.
Here’s an example of how to handle infinite scrolling using Selenium:
from selenium import webdriver
import time
# Initialize the webdriver
driver = webdriver.Chrome()
# URL of the page with infinite scrolling
url = "https://www.tiktok.com/discover/new"
driver.get(url)
# Keep scrolling until the desired amount of data is loaded
last_height = driver.execute_script("return document.body.scrollHeight")
while True:
# Scroll to the bottom of the page
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
# Wait for the page to load new content
time.sleep(2)
# Calculate the new scroll height and compare with the last height
new_height = driver.execute_script("return document.body.scrollHeight")
if new_height == last_height:
break
last_height = new_height
# Extract the loaded content
html = driver.page_source
# ... (parsing and data extraction code)
# Close the webdriver
driver.quit()
c. Data Parsing and Cleaning
Once you’ve extracted the raw HTML or JavaScript data, you’ll need to parse and clean it to retrieve the desired information. This may involve using regular expressions, handling inconsistencies in data formats, and removing irrelevant elements.
# Raw HTML data
html_data = "<div class='video-info'><h3 class='title'>Video Title</h3><span class='views'>1.2M views</span></div>"
# Extract video title using regular expressions
title_pattern = r"<h3 class='title'>(.*?)</h3>"
video_title = re.search(title_pattern, html_data).group(1)
# Extract view count and clean it
view_pattern = r"<span class='views'>(.*?) views</span>"
view_count = re.search(view_pattern, html_data).group(1)
view_count = view_count.replace(",", "")
print("Video Title:", video_title)
print("View Count:", view_count)
Scraping Different Types of TikTok Data
TikTok offers a wealth of data that can be scraped, including user profiles, video metadata, hashtags, comments, trending sounds, and more. Here’s an overview of scraping different types of TikTok data:
User Data (Profiles, Followers, Following)
Scraping user data can provide valuable insights into your target audience, influencers, and competitors. You can extract information such as usernames, bios, follower counts, location, and engagement metrics.
Video Data
1. Video Metadata (Titles, Descriptions, Hashtags, Views, Likes)
Video metadata includes essential information like titles, descriptions, hashtags, view counts, like counts, and more. This data can be used for trend analysis, content performance evaluation, and competitor research.
2. Downloading Video Content (Tools, Libraries, Copyright Considerations)
While downloading video content directly from TikTok may raise copyright concerns, there are some third-party tools and libraries that claim to facilitate video downloads. However, it’s essential to exercise caution and ensure compliance with TikTok’s policies and copyright laws.
Hashtag and Trend Data
1. Trending Hashtags and Challenges
Identifying trending hashtags and viral challenges can help you create timely and relevant content. By scraping hashtag data, you can uncover popular topics, analyze their performance, and tailor your content strategy accordingly.
2. Hashtag Performance and Engagement
In addition to trending hashtags, you can scrape data on hashtag performance, such as view counts, engagement rates, and top-performing videos using specific hashtags. This information can inform your hashtag strategy and content optimization efforts.
Comment and Engagement Data
1. Extracting Comments, Replies, and Sentiment
Scraping comment data can provide valuable insights into user sentiment, feedback, and engagement levels. You can extract comments, replies, usernames, timestamps, and even perform sentiment analysis on the collected data.
2. Engagement Metrics (Likes, Shares, Saves)
Engagement metrics, such as likes, shares, and saves, can help you evaluate the performance of your content or that of your competitors. By scraping this data, you can identify top-performing videos, analyze engagement patterns, and make data-driven decisions.
Sound and Music Data (Trending Sounds, Attribution)
TikTok is known for its viral sound trends, with users creating content around popular music snippets or audio clips. By scraping sound and music data, you can identify trending sounds, track their popularity, and attribute them to the original creators or rights holders.
Advanced Scraping Techniques
As TikTok’s anti-scraping measures evolve, more advanced techniques may be required to ensure successful and efficient data collection. Here are some advanced scraping techniques to consider:
Handling Captchas and Anti-Scraping Measures
TikTok employs various anti-scraping measures, such as captchas and bot detection mechanisms, to protect its platform from automated access. To overcome these challenges, you may need to implement techniques like headless browsing, image recognition, and human-in-the-loop solutions.
Implementing Proxies and IP Rotation
To avoid detection and potential IP bans, it’s often recommended to use proxies and rotate IP addresses during large-scale scraping operations. This can help distribute your requests across multiple IP addresses, reducing the risk of being flagged as a bot.
Scraping with Headless Browsers (Puppeteer, Playwright)
Headless browsers, such as Puppeteer (for Chrome) and Playwright (cross-browser), can be powerful tools for scraping dynamic content and handling JavaScript-heavy websites like TikTok. These tools simulate a real user’s browsing experience, making it harder for anti-scraping measures to detect your scraping activities.
Scraping at Scale (Parallelization, Distributed Scraping)
For large-scale scraping projects, you may need to employ techniques like parallelization and distributed scraping. Parallelization involves running multiple scraping processes simultaneously, while distributed scraping involves splitting the workload across multiple machines or servers. These techniques can significantly improve scraping efficiency and reduce the overall time required for data collection.
Working with Scraped Data
Once you’ve successfully scraped the desired data from TikTok, it’s essential to store, clean, and analyze it effectively.
Data Storage (Databases, JSON, CSV)
The choice of data storage format will depend on the size and complexity of your scraped data. For smaller datasets, you can store data in CSV or JSON files. For larger datasets or more complex data structures, you may want to consider using a database management system like MySQL, PostgreSQL, or MongoDB.
Data Cleaning and Preprocessing
Raw scraped data often contains inconsistencies, duplicates, or irrelevant information. Data cleaning and preprocessing are crucial steps to ensure the quality and usability of your data. This may involve tasks like removing HTML tags, handling missing values, normalizing data formats, and deduplicating records.
Data Analysis and Visualization (Python Libraries)
Once your data is cleaned and organized, you can leverage Python’s powerful data analysis and visualization libraries to derive insights. Libraries like Pandas, NumPy, and Matplotlib can help you perform data manipulation, statistical analysis, and create informative visualizations like charts, graphs, and heat maps.
Generating Insights and Reports
The ultimate goal of data scraping is to generate actionable insights and reports that can inform business decisions or support research efforts. Based on your analysis, you can create reports highlighting key findings, trends, and recommendations tailored to your specific use case.
Troubleshooting Common Scraping Issues
Despite your best efforts, you may encounter various issues during the scraping process. Here are some common problems and potential solutions:
1. Getting Blocked or Banned by TikTok
If TikTok detects your scraping activities, they may temporarily or permanently block your IP address or implement other anti-scraping measures. To mitigate this issue, you can:
- Implement rotating proxies or use a proxy service provider to distribute your requests across multiple IP addresses.
- Adjust your scraping speed and implement appropriate delays between requests to avoid overwhelming TikTok’s servers.
- Randomize your user agent strings to mimic real user behavior.
- Use headless browsers or tools like Selenium to simulate human-like interactions with the website.
2. Handling JavaScript-Rendered Content
Some parts of TikTok’s website may rely on JavaScript to render content dynamically. If you’re using a library like BeautifulSoup, which parses static HTML, you may not be able to extract this data. To handle dynamic content, you can:
- Use a tool like Selenium, which can execute JavaScript and interact with the website as a real user would.
- Explore alternative libraries like Splash or PyppeteerChromium, which can render JavaScript-heavy pages and extract the rendered content.
3. Dealing with Infinite Scrolling or Pagination
TikTok’s website may implement infinite scrolling or pagination to load more content as the user scrolls or clicks a “Load More” button. To scrape data from these pages, you’ll need to:
- Identify the patterns or triggers that load additional content (e.g., scrolling to the bottom of the page, clicking a button).
- Use tools like Selenium to simulate these actions and wait for the new content to load before extracting data.
- Implement logic to check if new content has been loaded and stop scraping when no more data is available.
4. Parsing and Cleaning Inconsistent Data
The data you scrape from TikTok may sometimes be inconsistent or contain irrelevant information. To handle this, you can:
- Use regular expressions and string manipulation techniques to clean and normalize the data.
- Implement data validation checks to identify and handle missing or invalid data.
- Explore libraries like Pandas or NumPy for advanced data cleaning and preprocessing capabilities.
5. Handling Captchas and Other Anti-Scraping Measures
TikTok may employ captchas or other anti-scraping measures to prevent automated access. While solving captchas programmatically can be challenging, you can consider the following approaches:
- Implement image recognition techniques using libraries like OpenCV or Tesseract to solve simple captchas.
- Use captcha-solving services or APIs that leverage human or machine learning solutions.
- Implement a hybrid approach where you automatically handle known captcha types and involve human intervention for more complex cases.
Remember, troubleshooting is an iterative process, and you may need to adjust your scraping techniques based on the challenges you encounter and the evolving anti-scraping measures implemented by TikTok.